Tasks and their execution
Task is a job prepared for execution.
Contents:
Classes
Classes required to execute the job reside in the ppc_robot_lib.tasks
module.
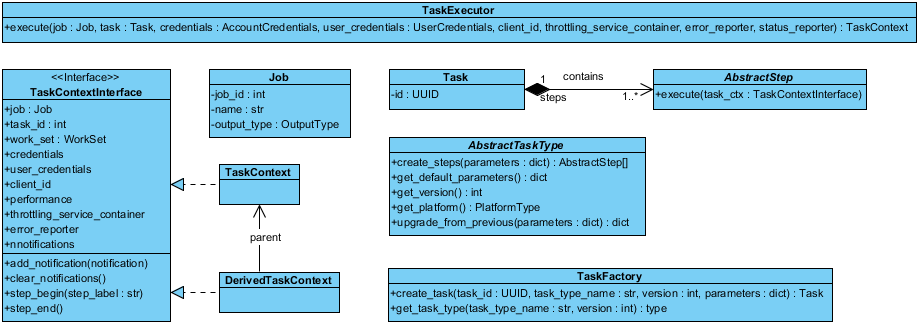
- class Task(task_id, task_level, steps=None, report_function=None, parameters=None)[source]
Represents a currently executing task (instance of job).
- property parameters: dict[str, Any]
- Returns:
Report parameters that should be passed to the
report_function
.
- property report_function: Callable[[dict[str, Any]], None]
- Returns:
Function that should be called in order to run the report.
- property steps: Iterable[AbstractStep]
- Returns:
List of task steps.
- property uses_function_execution_model
- Returns:
Whether the task should use the new function execution model.
- class AbstractTaskType[source]
Base class for task types.
- classmethod convert_parameters(data)[source]
Convert parameters from dictionary to an object which can be passed to
execute()
orcreate_steps()
.
- classmethod convert_upgrade_parameters(data)[source]
Convert parameters from dictionary to an object which can be passed to
upgrade_from_previous()
.
- abstract create_steps(parameters)[source]
Creates steps which must be completed during execution of the task.
- Parameters:
- Return type:
- Returns:
Steps for the Task.
- abstract classmethod get_name()[source]
Gets the internal task type name. Do not include the platform name!
Incorrect: adwords_custom_report
Correct: custom_report
- Return type:
- Returns:
Internal task type name.
- classmethod get_parameters_class()[source]
Determines class which should be used to represent task parameters. The default implementation will return the class used as type hint for
parameters
argument in theexecute()
method (or in thecreate_steps()
, if the task does not use the functional execution model).
- abstract classmethod get_platform()[source]
Gets the platform that is used by this type.
- Return type:
PlatformType
- Returns:
Platform constant.
- classmethod get_previous_parameters_class()[source]
Determines class which should be used to represent task parameters. The default implementation will return the class used as type hint for
parameters
argument in theupgrade_from_previous()
method.
- abstract classmethod get_version()[source]
Gets the version number
- Return type:
- Returns:
Version as positive integer.
- task_kind = 'report'
Defines kind of this task type: report or robot.
- abstract classmethod upgrade_from_previous(parameters)[source]
Upgrades the set of parameters from previous version to this version. Will be called when user creates a job and then a new version of that job type is introduced. It needs to handle only upgrade from previous version. See
ppc_robot_lib.tasks.task_type_versions.TaskTypeVersions.upgrade_to_latest()
for more details about the process of parameters upgrade.If this is the first version, simply return the
parameters
to make all static checkers happy.
- uses_function_execution_model = False
Defines whether the task type uses functional or steps-based model.
- classmethod validate(previous_version_cls)[source]
Validates that the report class is defined correctly.
Performs the following validations: * The type passed to
upgrade_from_previous()
matches the parameters type reported by the previous version.- Parameters:
previous_version_cls (
type
[AbstractTaskType
] |None
) – Class of the previous report version.None
if there is no previous version.- Return type:
- class AbstractFunctionTaskType[source]
Base class for all task types that uses the function execution model. This class should not be subclassed directly, use either
AbstractReportType
orAbstractRobotType
.- abstract execute(parameters)[source]
Called when the report is executed. This method must be implemented in the subclasses.
- uses_function_execution_model = True
Defines whether the task type uses functional or steps-based model.
- class AbstractReportType[source]
- task_kind = 'report'
Defines kind of this task type: report or robot.
- class AbstractRobotType[source]
- task_kind = 'robot'
Defines kind of this task type: report or robot.
- class TaskTypeVersions(versions)[source]
Descriptor that contains all versions of the given task type. When this class is instantiated, it goes through all versions and builds a list. The versions does not necessarily need to be linear sequence, the only rules are:
version numbers must be unique, and
newer version has a higher number.
- Parameters:
versions (
list
[type
[AbstractTaskType
]]) – List of all task type versions.
- get_task_type(version)[source]
- Parameters:
version (
int
) – Version number.- Return type:
- Returns:
Task type in the given version.
- upgrade_to_latest(parameters, version)[source]
Upgrades the given parameters from the given version to latest version.
Calls
AbstractTaskType.upgrade_from_previous
on all versions betweenversion
and the latest version – if the job definition needs to be upgraded for multiple versions, they are called for each version – e.g. if version 2 is being upgraded to version 5,upgrade_from_previous
is called for version 3, 4 and 5, in that order.If the task already is in the last version, nothing is done.
- class TaskFactory(task_id_allocator=None)[source]
Creates task from job definition. Contains dictionary of named task types.
- Parameters:
task_id_allocator (
TaskIdAllocator
|None
) – Deprecated service for allocating task IDs. Never used, kept for backwards compatibility.
- create_task(task_id, platform, task_type_name, version, parameters)[source]
Creates a task of given type with given parameters. :type task_id:
UUID
:param task_id: Assigned Task ID. If not given, a new one is generated. :type platform:PlatformType
:param platform: Platform. :type task_type_name:str
:param task_type_name: Name of the task type. :type version:int
:param version: Task type version :type parameters:dict
[str
,Any
] :param parameters: Task parameters. :return: Created task.
- get_task_type(platform, task_type_name, version)[source]
Gets task type with given name. :type platform:
PlatformType
:param platform: Platform. :type task_type_name:str
:param task_type_name: Name of the task type. :type version:int
:param version: Task type version. :rtype:type
[AbstractTaskType
] :return: Task type.
- class TaskExecutor(context_factory)[source]
Executes tasks with the given account credentials: creates a working set, prepares context and iterates through task steps and executes them.
- class TaskContextInterface[source]
Interface describing the task context, which is passed to each step during its execution.
- add_metric_card(metric_card)[source]
Adds a new metric card to current job. These cards will be saved if the job completes successfully.
- Parameters:
metric_card (ppc_robot_lib.models.JobMetricCard) – Metric card to add.
- Return type:
- abstract add_notification(notification)[source]
Adds a new notification.
- Parameters:
notification (ppc_robot_lib.models.Notification) – Notification to add.
- abstract property client_id: Any
- Returns:
Client ID of Client Account. Can be null for service account-level jobs.
- abstract property client_login_id: Any
- Returns:
Login Client ID of Client Account. Can be null for service account-level jobs.
- create_derived_ctx(target_object)[source]
Creates a new derived context.
- Parameters:
target_object (
TargetObject
) – Object that will be set as target in the derived context.- Return type:
- Returns:
New derived context that inherits from the current context.
- abstract property credentials: AccountCredentials
- Returns:
Credentials for the given Service Account.
- abstract property currency: str | None
- Returns:
Currency code of Client Account. Can be null for service account-level jobs.
- abstract property error_reporter: Callable[[Exception], None]
- Returns:
Callable that stores or logs an exception that might occur during the execution.
- abstract property job: Job
- Returns:
Job descriptor.
- abstract property performance: TaskPerformance
- Returns:
Performance metrics for the whole task.
- abstract step_begin(step_label)[source]
Should be called by the executor when a step is about to begin.
Can be used for reporting of the current state. Implementation should handle nesting of steps and store the full stack.
- Parameters:
step_label (
str
) – String representation of the current step.
- abstract step_end()[source]
Should be called by the executor when a step has ended. It has to be paired with call to
step_begin()
.
- abstract property throttling_service_container: ServiceContainerInterface
- Returns:
Optional container that handles throttling of various services.
- abstract property user_credentials: UserCredentials
- Returns:
OAuth2 credentials of the user – can be used to access his Google Drive.
- abstract property work_set: WorkSet
- Returns:
WorkSet of the task that is mutated during the execution.
- class TaskContext(job, task_id, work_set, credentials, user_credentials, locale=None, client_id=None, currency=None, throttling_service_container=None, error_reporter=None, status_reporter=None, client_login_id=None, target_object=None, context_factory=None)[source]
Class used to store context of the currently executing task. The same instance is passed to each step.
- add_metric_card(metric_card)[source]
Adds a new metric card to current job. These cards will be saved if the job completes successfully.
- Parameters:
metric_card (ppc_robot_lib.models.JobMetricCard) – Metric card to add.
- Return type:
- add_notification(notification)[source]
- Parameters:
notification (ppc_robot_lib.models.Notification) – Notification to add.
- property client_id: Any
- Returns:
Client ID of Client Account. Can be null for service account-level jobs.
- property client_login_id: Any
- Returns:
Login Client ID of Client Account. Can be null for service account-level jobs.
- property currency: str
- Returns:
Currency code of Client Account. Can be null for service account-level jobs.
- property error_reporter: Callable[[Exception], None]
- Returns:
Callable that stores or logs an exception that might occur during the execution.
- property job: Job
- Returns:
Job ID.
- property performance: TaskPerformance
- Returns:
Performance metrics for the whole task.
- step_begin(step_label)[source]
Should be called by the executor when a step is about to begin.
Can be used for reporting of the current state. Implementation should handle nesting of steps and store the full stack.
- Parameters:
step_label (
str
) – String representation of the current step.
- step_end()[source]
Should be called by the executor when a step has ended. It has to be paired with call to
step_begin()
.
- property throttling_service_container: ServiceContainerInterface
- Returns:
Optional container that handles throttling of various services.
- property user_credentials
- Returns:
OAuth2 credentials of the user – can be used to access his Google Drive.
- property user_locale
- Returns:
User’s locale.
- property work_set: WorkSet
- Returns:
Work Set.
- class DerivedTaskContext(parent, work_set, client_id=None, currency=None, client_login_id=None, target_object=None, credentials=None)[source]
Represents a derived context. Shares job ID, task ID and credentials with the parent context and has it’s own work set and client ID.
- add_metric_card(metric_card)[source]
Adds a new metric card to current job. These cards will be saved if the job completes successfully.
- Parameters:
metric_card (ppc_robot_lib.models.JobMetricCard) – Metric card to add.
- Return type:
- add_notification(notification)[source]
Adds a new notification.
- Parameters:
notification (ppc_robot_lib.models.Notification) – Notification to add.
- property client_id: Any
- Returns:
Client ID of Client Account. Can be null for service account-level jobs.
- property client_login_id: Any
- Returns:
Login Client ID of Client Account. Can be null for service account-level jobs.
- property credentials: AccountCredentials
- Returns:
Credentials for the given Service Account.
- property currency: str
- Returns:
Currency code of Client Account. Can be null for service account-level jobs.
- property error_reporter: Callable[[Exception], None]
- Returns:
Callable that stores or logs an exception that might occur during the execution.
- property job: Job
- Returns:
Job descriptor.
- property notifications
- Returns:
List of all notifications.
- property performance
- Returns:
Performance metrics for the whole task.
- step_begin(step_label)[source]
Should be called by the executor when a step is about to begin.
Can be used for reporting of the current state. Implementation should handle nesting of steps and store the full stack.
- Parameters:
step_label (
str
) – String representation of the current step.
- step_end()[source]
Should be called by the executor when a step has ended. It has to be paired with call to
step_begin()
.
- property throttling_service_container: ServiceContainerInterface
- Returns:
Optional container that handles throttling of various services.
- property user_credentials
- Returns:
OAuth2 credentials of the user – can be used to access his Google Drive.
- property user_locale
- Returns:
User’s locale.
- property work_set: WorkSet
- Returns:
WorkSet of the task that is mutated during the execution.